Commits on Source (17)
-
Rhys Nute authorede634c996
-
Rhys Nute authoredcf741af1
-
Rhys Nute authored8a04aee4
-
Rhys Nute authored5296b67e
-
Rhys Nute authored56c33c55
-
Rhys Nute authored3d96591a
-
Rhys Nute authorede63d275a
-
Rhys Nute authoredf9265540
-
Rhys Nute authoredbacc9723
-
Rhys Nute authoreda1bd0569
-
Rhys Nute authored264db9d2
-
Rhys Nute authorede9129acb
-
Rhys Nute authored49efe83d
-
Rhys Nute authored1e184fbc
-
Rhys Nute authored15f288c0
-
Rhys Nute authored3d3cc9dc
-
Rhys Evans authored
Ready to merge - Database Closes #30 See merge request !16
cd693090
Showing
- build.gradle 3 additions, 2 deletionsbuild.gradle
- identifier.sqlite 0 additions, 0 deletionsidentifier.sqlite
- src/main/java/Team5.Smarttowns/Data/Towns.db 0 additions, 0 deletionssrc/main/java/Team5.Smarttowns/Data/Towns.db
- src/main/java/Team5/SmartTowns/Data/DatabaseController.java 40 additions, 0 deletionssrc/main/java/Team5/SmartTowns/Data/DatabaseController.java
- src/main/java/Team5/SmartTowns/Data/UserRepository.java 8 additions, 0 deletionssrc/main/java/Team5/SmartTowns/Data/UserRepository.java
- src/main/java/Team5/SmartTowns/Data/UserRepositoryJDBC.java 36 additions, 0 deletionssrc/main/java/Team5/SmartTowns/Data/UserRepositoryJDBC.java
- src/main/java/Team5/SmartTowns/Data/location.java 12 additions, 0 deletionssrc/main/java/Team5/SmartTowns/Data/location.java
- src/main/java/Team5/SmartTowns/Data/locationRepository.java 10 additions, 0 deletionssrc/main/java/Team5/SmartTowns/Data/locationRepository.java
- src/main/java/Team5/SmartTowns/Data/locationRepositoryJDBC.java 29 additions, 0 deletions...in/java/Team5/SmartTowns/Data/locationRepositoryJDBC.java
- src/main/java/Team5/SmartTowns/Data/trail.java 12 additions, 0 deletionssrc/main/java/Team5/SmartTowns/Data/trail.java
- src/main/java/Team5/SmartTowns/Data/trailsRepository.java 8 additions, 0 deletionssrc/main/java/Team5/SmartTowns/Data/trailsRepository.java
- src/main/java/Team5/SmartTowns/Data/trailsRepositoryJDBC.java 28 additions, 0 deletions...main/java/Team5/SmartTowns/Data/trailsRepositoryJDBC.java
- src/main/java/Team5/SmartTowns/Data/user.java 12 additions, 0 deletionssrc/main/java/Team5/SmartTowns/Data/user.java
- src/main/resources/QRCodeScanner.html 10 additions, 0 deletionssrc/main/resources/QRCodeScanner.html
- src/main/resources/application.properties 4 additions, 0 deletionssrc/main/resources/application.properties
- src/main/resources/data.sql 11 additions, 0 deletionssrc/main/resources/data.sql
- src/main/resources/schema.sql 18 additions, 0 deletionssrc/main/resources/schema.sql
- src/main/resources/static/images/Facebook.png 0 additions, 0 deletionssrc/main/resources/static/images/Facebook.png
- src/main/resources/static/images/Instagram.jpg 0 additions, 0 deletionssrc/main/resources/static/images/Instagram.jpg
- src/main/resources/static/images/LinkedIn.jpg 0 additions, 0 deletionssrc/main/resources/static/images/LinkedIn.jpg
identifier.sqlite
0 → 100644
src/main/java/Team5.Smarttowns/Data/Towns.db
0 → 100644
File added
src/main/resources/QRCodeScanner.html
0 → 100644
src/main/resources/data.sql
0 → 100644
src/main/resources/schema.sql
0 → 100644
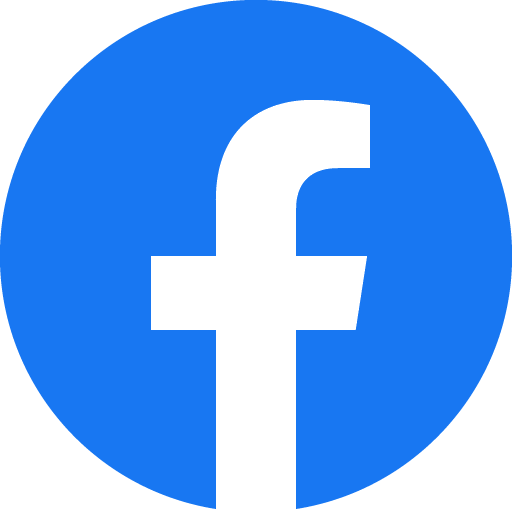
| W: | H:
| W: | H:
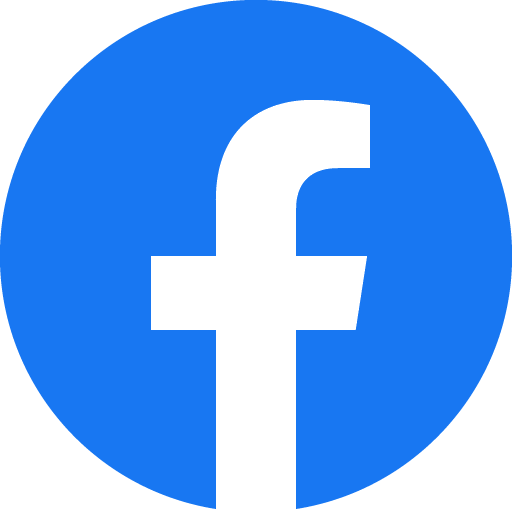
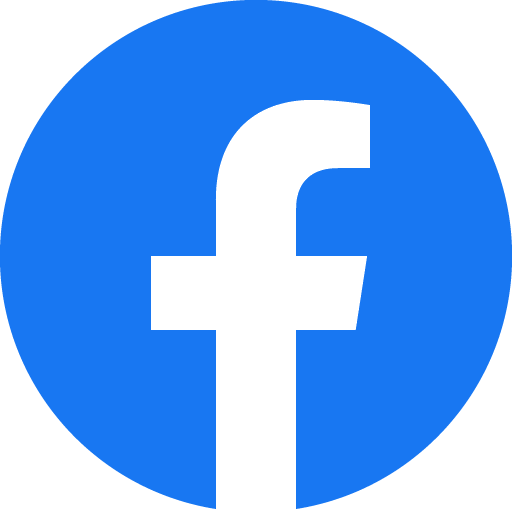

| W: | H:
| W: | H:


34.7 KiB